[MainProject] Footer
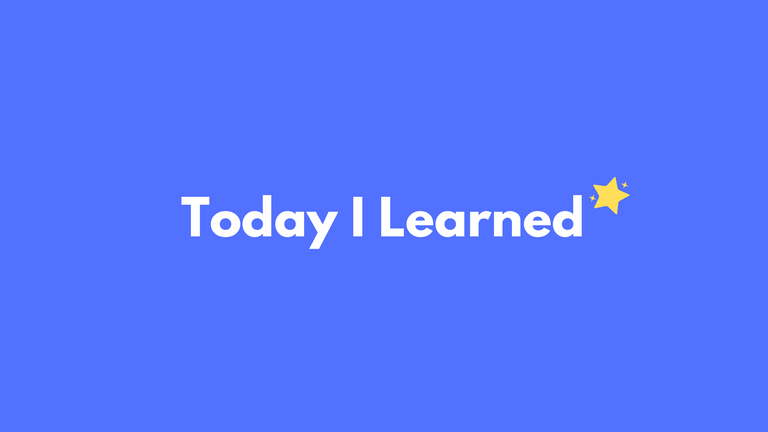
Footer
웹 페이지에서 공통 컴포넌트로 사용되는 Footer
구현
Footer style
styled-components를 사용하여 Footer 컴포넌트의 스타일을 정의
//Footer.style.ts
import styled from "styled-components";
export const FooterContainer = styled.div`
position: relative;
display: flex;
flex-direction: column;
justify-content: center;
align-items: center;
background-color: var(--purple);
width: 100%;
color: var(--color-white);
font-family: var(--font-noto-sans-kr);
margin-top: auto;
`;
export const FooterInfoContainer = styled.div`
display: flex;
justify-content: space-around;
width: 40%;
margin-bottom: 8px;
@media (max-width: 1000px) {
font-size: 14px;
margin-top: 24px;
width: 100%;
}
`;
export const InfoBlock = styled.div`
display: flex;
flex-direction: column;
align-items: flex-start;
margin-left: 70px;
@media (max-width: 1000px) {
font-size: 12px;
margin-left: 50px;
}
`;
export const RightInfoBlock = styled.div`
display: flex;
flex-direction: column;
align-items: flex-start;
@media (max-width: 1000px) {
font-size: 12px;
}
`;
export const ImageContainer = styled.div`
display: flex;
justify-content: space-between;
width: 12%;
@media (max-width: 1000px) {
width: 50%;
}
`;
FooterContainer: Footer의 전체 컨테이너 스타일을 정의합니다. 배경색, 글꼴, 정렬 등의 스타일을 지정
FooterInfoContainer: Footer 내 정보 블록들을 감싸는 컨테이너의 스타일을 정의합니다. 미디어 쿼리를 활용하여 화면 크기에 따른 스타일 변화를 처리
InfoBlock, RightInfoBlock: Footer 내 정보 블록들의 스타일을 정의합니다. 정렬, 여백, 글꼴 크기 등을 지정하며, 미디어 쿼리를 사용하여 반응형 스타일을 구현
ImageContainer: 아이콘 이미지들을 담는 컨테이너의 스타일을 정의합니다. 마찬가지로 미디어 쿼리를 활용하여 반응형 스타일을 처리
Footer 컴포넌트
styled-components를 사용하여 Footer 컴포넌트의 스타일을 정의
import { useEffect, useState } from "react";
import { useLocation } from "react-router-dom";
import github from "../assets/images/github.png";
import notion from "../assets/images/notion.png";
import youtube from "../assets/images/youtube.png";
import { BASE_ANIMATION_TIME } from "../assets/constantValue/constantValue";
import {
FooterContainer,
FooterInfoContainer,
InfoBlock,
RightInfoBlock,
ImageContainer,
} from "./Footer.style";
function Footer() {
// 현재 경로 정보를 가져오는 useLocation 훅 사용
const location = useLocation();
// 초기 애니메이션 상태 설정 함수
const initialAnimationState = () => {
if (location.pathname === "/auth") {
return "none";
}
return "fadeIn";
};
// 애니메이션 상태를 관리하는 useState 훅
const [animation, setAnimation] = useState(initialAnimationState);
// 경로 변화에 따라 애니메이션 상태를 변경하는 useEffect 훅
useEffect(() => {
// 경로가 '/auth' 또는 '/' 인 경우 fadeIn 애니메이션 적용
if (
(location.pathname === "/auth" || location.pathname === "/") &&
animation === "fadeIn"
) {
// 일정 시간 후에 애니메이션 클래스 제거 (fadeOut)
setTimeout(() => setAnimation("none"), BASE_ANIMATION_TIME);
setAnimation("fadeOut");
return;
}
// 다른 경로인 경우 fadeIn 애니메이션 적용
if (location.pathname !== "/auth" && location.pathname !== "/") {
setTimeout(() => setAnimation("fadeIn"), BASE_ANIMATION_TIME);
return;
}
}, [location]);
return (
<FooterContainer className={`${animation}`}>
{/* 상단 로고 부분 */}
<div
style=
>
BUYTE
</div>
{/* 정보 블록 컨테이너 */}
<FooterInfoContainer>
{/* 왼쪽 정보 블록 */}
<InfoBlock>
<div
style=
>
(주) BUYTE
</div>
<div style=>010-1234-1234</div>
</InfoBlock>
{/* 오른쪽 정보 블록 */}
<RightInfoBlock>
<div
style=
>
22팀 | BUYTE 팀 메인프로젝트{" "}
</div>
<div
style=
>
FE : 김준표 | 민정호 | 양효정{" "}
</div>
<div style=>
BE : 김현우 | 오숙현 | 이준기
</div>
</RightInfoBlock>
</FooterInfoContainer>
{/* 아이콘 이미지 컨테이너 */}
<ImageContainer>
{/* GitHub 아이콘 링크 */}
<a href="https://github.com/codestates-seb/seb44_main_022">
<img
src={github}
alt="GitHub"
style=
/>
</a>
{/* Notion 아이콘 */}
<img
src={notion}
alt="Notion"
style=
/>
{/* YouTube 아이콘 */}
<img
src={youtube}
alt="YouTube"
style=
/>
</ImageContainer>
</FooterContainer>
);
}
export default Footer;
useLocation: useLocation
훅을 사용하여 현재 경로 정보를 가져온다. 이 정보를 활용하여 경로에 따른 애니메이션 상태를 관리한다.
initialAnimationState: 초기 애니메이션 상태를 설정하는 함수 만약 경로가 /auth
인 경우에는 애니메이션 없음을 의미하는 ‘none’을 반환하고, 그 외의 경우에는 fadeIn 애니메이션을 의미하는 fadeIn
을 반환한다.
useState: 초기 애니메이션 상태를 initialAnimationState의
반환 값으로 설정하고, setAnimation
함수를 받아 애니메이션 상태를 변경한다.
useEffect: 경로 변화를 감지하여 애니메이션 상태를 변경하는 부분 경로가 /auth 또는 /
인 경우에는 fadeIn
애니메이션 적용 후 일정 시간이 지난 뒤에 fadeOut 애니메이션 적용합니다. 그 외의 경우에는 fadeIn
애니메이션을 적용한다.
구현 이미지
댓글남기기